You’d think adding a modal into a NextJS project would be easy. Just import BootStrap, create a button or link with the modal ID, slap the modal on a page and voila you’re done. But there’s more to it than just that.
Displaying content in a modal utilizes routing, which is how NextJS navigates pages.
Considering that you’re already familiar with Parallel and Intercepting* Routes, let’s take a look at creating modals.
Let’s take a look at the app.
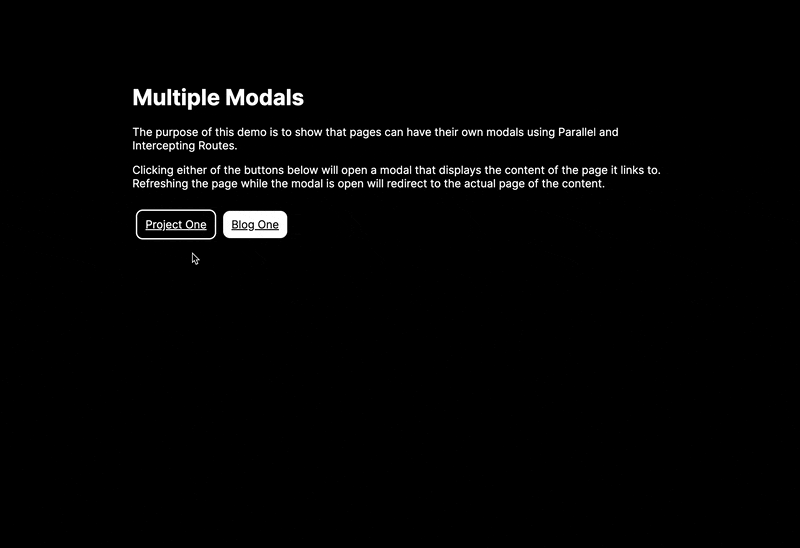
DEMO: https://multiple-modals.vercel.app/
The main page has two buttons that lead to an individual Project page and Blog page. The goal is to display them as a modal instead of directly going to them.
First, let’s make a singular modal in an existing NextJS app.
Part one: set up the parallel route
1. Create a new folder in the app folder and name it “@modal”. The @ symbol signifies that a parallel route is in place.
2. Within this folder, create a default.js file.
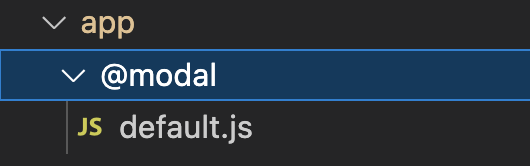
3. The default file will return a null component.
export default function ModalDefaultPage() {
return null;
}
Part two: set up the intercepting route
4. Create a new folder within the @modal folder and give it the same name of the page you’re intercepting. Add (.) in front of the name.
The (.) helps define the page you want to match as an intercepted route. This is similar to relative pathing.
So if we intercept a page one level above, the signifier will be (..)
Two levels above will be (..)(..)
Matching the root/app will be (…)
In this case, we’re creating a folder called (.)projects. Also, since we’re targeting dynamic routes, we will also copy the original file structure.
So far, the @modal structure should be as follows:
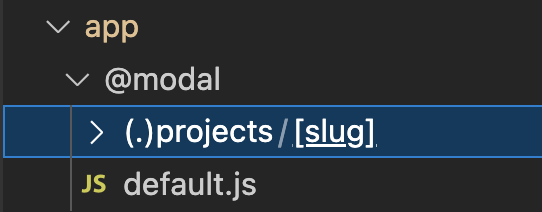
5. Within this folder, create a page.js file containing the modal view you want to display.
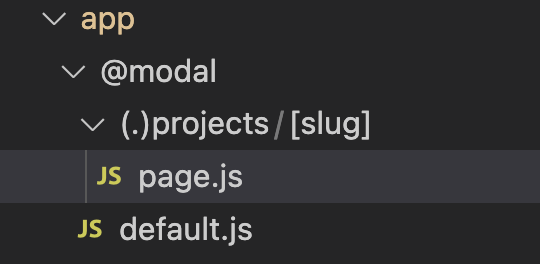
This could be similar to your original page’s design. The major difference will be that the content appears in a modal.
6. Within the root layout.js file, bring in @modal as a prop and place it in your code.
export default function RootLayout({ children, modal }) {
return (
<html lang="en">
<body className={inter.className}>
{children}
{modal}
</body>
</html>
);
}
Adding more modals
To create more modals, you will repeat steps 4 – 5. Continue using the same parallel folder (@modal) and add additional intercepting folders for each page you want to make it for.
Since we’re reusing the parallel modal route, we don’t need to modify the layout file again as we did in step 6.
*This YouTube video explains Intercepting Modals in a way simpler than the NextJS docs.